Quick Start
MiniKit-JS SDK
MiniKit-JS is our official SDK for creating mini apps that work with World App. It provides handy functions and types to make development a breeze.
Quick Start
The fastest way to get started is by using our template next-15 repository.
Run the following command and follow the instructions to create a new mini app. For cleanliness we recommend using pnpm
as your package manager.
npx @worldcoin/create-mini-app my-first-mini-app
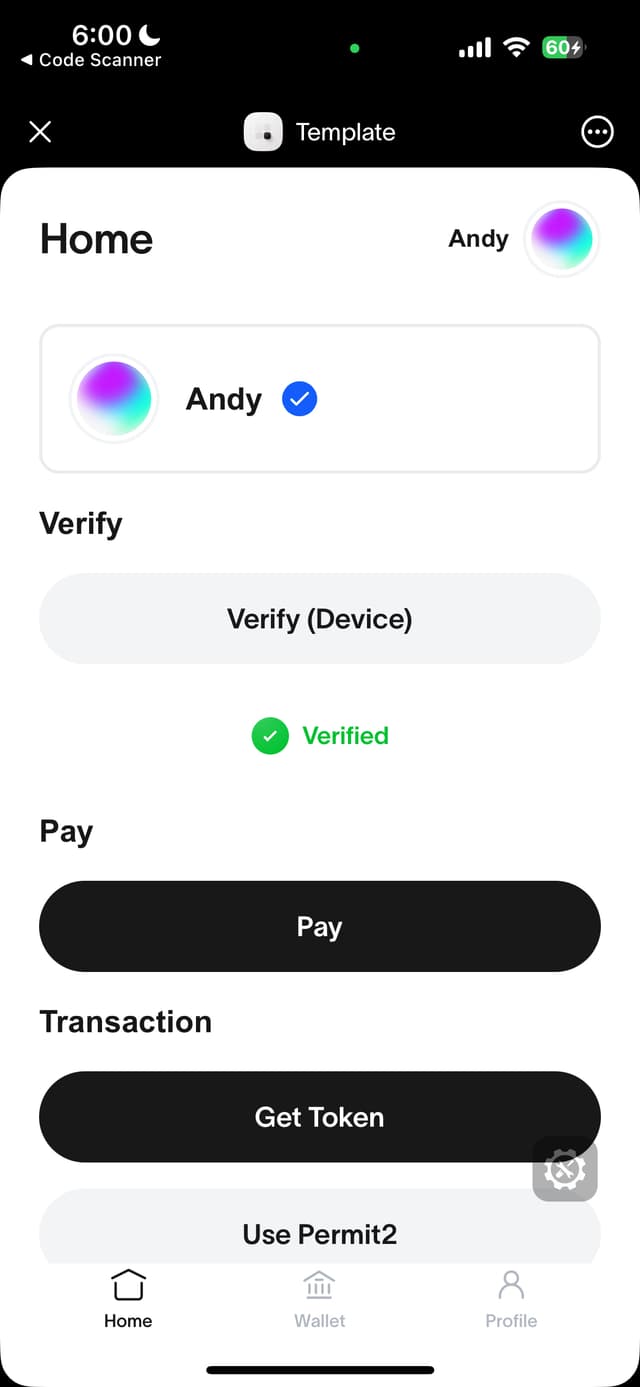
Correctly running template should look like this
Manual Installation
MiniKit-JS is the core lib, framework agnostic,
MiniKit-React provides hooks that make it easy to interact with the MiniKit SDK.
pnpm install @worldcoin/minikit-js
Or use a CDN like jsdelivr, for inline HTML, make sure to fill in the version.
<script>
import { MiniKit } from "https://cdn.jsdelivr.net/npm/@worldcoin/minikit-js@[version]/+esm"
</script>
Usage
- Wrap your root with the MiniKitProvider component.This provides the MiniKit object to your app.
src/index.tsx
import { MiniKitProvider } from '@worldcoin/minikit-js/minikit-provider';
export default async function Root({
children,
}: Readonly<{
children: React.ReactNode
}>) {
return (
<html lang="en">
<MiniKitProvider>
<body className={inter.className}>{children}</body>
</MiniKitProvider>
</html>
)
}
- Check if MiniKit is installed and ready to use
MiniKit.isInstalled()
will only return true if the app is opened and properly initialized inside the World App. This is useful to distinguish between a user opening your app in the browser or in the World App.
// ...
console.log(MiniKit.isInstalled())
Template Repositories
The following template repositories are also available:
- Vanilla JS (using a CDN) template (featuring a simple backend for verifications),
- Wallet Auth + Send Transaction example.
- Community example - Wallet Auth using JWT.
- Community example - Wallet Auth using NextAuth.
Otherwise, continue below with the installation instructions.
Watch a video tutorial here.