Quick Actions
World Chat Quick Action
World Chat is a messaging platform in the World App ecosystem. Quick Actions let you link to specific chat features.
Url follows the schema below.
https://worldcoin.org/mini-app?app_id=app_e293fcd0565f45ca296aa317212d8741
Parameters
- Name
username
- Type
- string
- Required
- REQUIRED
- Description
The username of the recipient you want to chat with. You can resolve a username using the user's wallet address.
- Name
message
- Type
- string
- Description
Predefined message text to include in the draft chat.
- Name
pay
- Type
- string
- Description
When included, opens a draft chat with the send payment option pre-selected. You can optionally specify an amount by using
pay=amount
where amount is the numeric value in USDC.e.
- Name
request
- Type
- string
- Description
When included, opens a draft chat with the payment request option pre-selected. You can optionally specify an amount by using
request=amount
where amount is the numeric value in USDC.e.
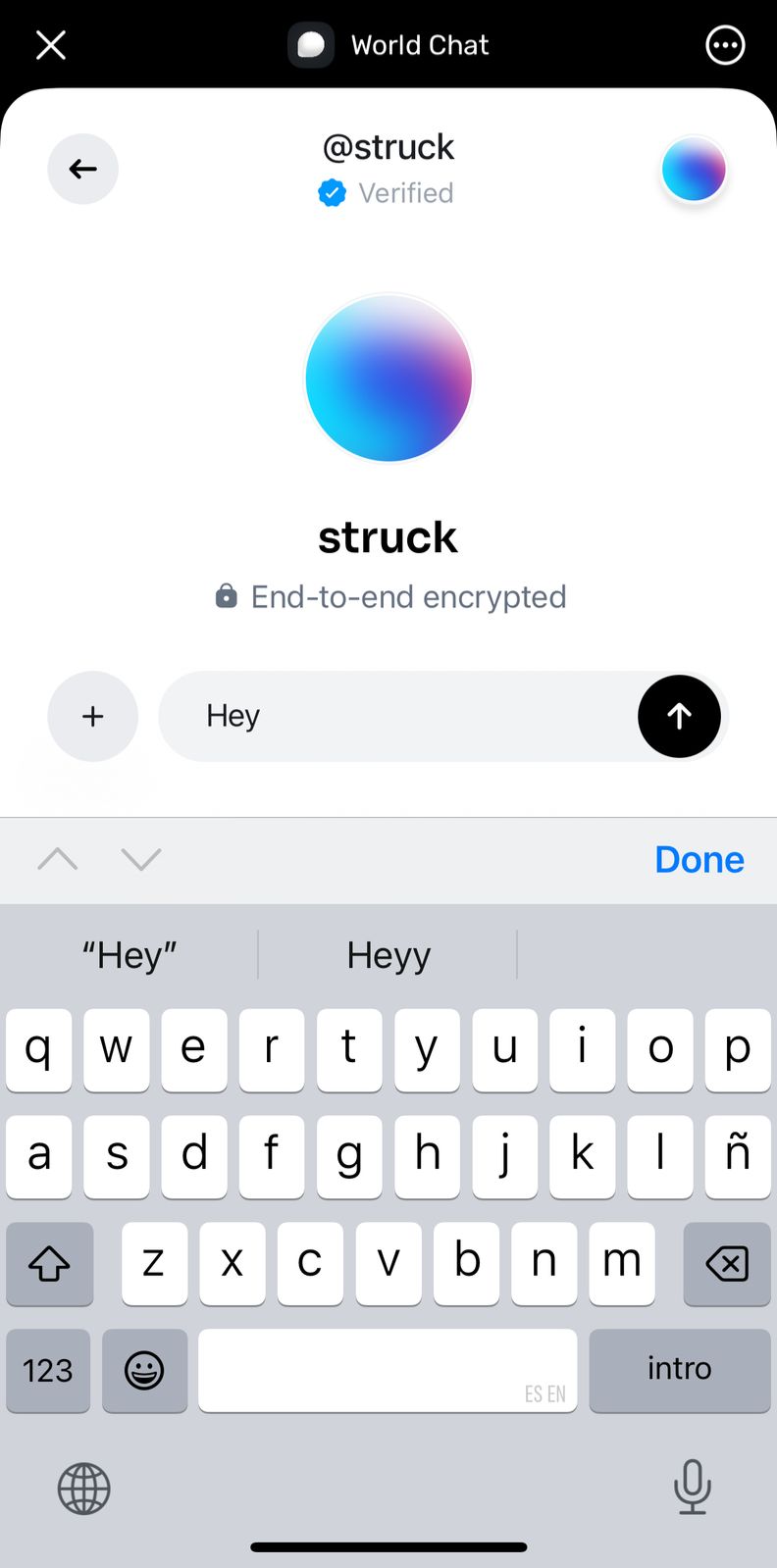
Helper function
const WORLD_CHAT_APP_ID = 'app_e293fcd0565f45ca296aa317212d8741'
function getWorldChatDeeplinkUrl({
username,
message,
pay,
request,
}: {
username: string
message?: string
pay?: string | number
request?: string | number
}) {
let path = `/${username}/draft`
if (message) {
path += `?message=${message}`
} else if (pay !== undefined) {
if (pay === 'true' || pay === true) {
path += `?pay`
} else {
path += `?pay=${pay}` // Pay with amount
}
} else if (request !== undefined) {
if (request === 'true' || request === true) {
path += `?request`
} else {
path += `?request=${request}` // Request with amount
}
}
const encodedPath = encodeURIComponent(path)
return `https://worldcoin.org/mini-app?app_id=${WORLD_CHAT_APP_ID}&path=${encodedPath}`
}
// Create a chat with predefined message
console.log(
getWorldChatDeeplinkUrl({
username: 'johndoe',
message: 'Hello from my mini app!',
})
)
// Create a chat with send payment option
console.log(
getWorldChatDeeplinkUrl({
username: 'johndoe',
pay: 'true',
})
)
// Create a chat with send payment option and amount
console.log(
getWorldChatDeeplinkUrl({
username: 'johndoe',
pay: 5.25,
})
)
// Create a chat with payment request option
console.log(
getWorldChatDeeplinkUrl({
username: 'johndoe',
request: 'true',
})
)
// Create a chat with payment request option and amount
console.log(
getWorldChatDeeplinkUrl({
username: 'johndoe',
request: 10,
})
)
Example output links
// Message draft
https://worldcoin.org/mini-app?app_id=app_e293fcd0565f45ca296aa317212d8741&path=%2Fjohndoe%2Fdraft%3Fmessage%3DHello
// Payment draft with amount
https://worldcoin.org/mini-app?app_id=app_e293fcd0565f45ca296aa317212d8741&path=%2Fjohndoe%2Fdraft%3Fpay%3D5.25
// Request draft with amount
https://worldcoin.org/mini-app?app_id=app_e293fcd0565f45ca296aa317212d8741&path=%2Fjohndoe%2Fdraft%3Frequest%3D10
Appendix
Caveats/Warnings
- The username must be a valid World ID username.
- Only one quick action type (message, pay, or request) can be used at a time.
- If the recipient does not exist, the app will show an appropriate error message.
- Only USDC.e & WLD are supported for payments.
- Currency amounts should be specified as decimal numbers (e.g., 5.25 for 5.25 USDC.e).